Animated Penguin Animated Old Fashioned Plane
sponsor
Code with Andrea is free for anybody. Help me keep it that way past checking out this sponsor:
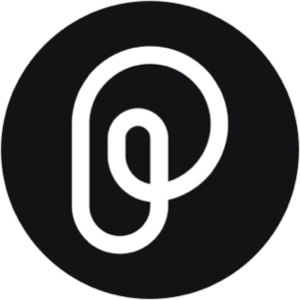
Save useful snippets straight from your IDE or browser. Extract code from screenshots. Pieces is the fastest way to heave your productivity and stay in flow.
Set up? Let'southward get started!
Implicitly Animated Widgets
Flutter ships with a bunch of so-chosen implicitly animated widgets that you just driblet in your code to easily add animations.
For example, let's take a look at AnimatedContainer.
AnimatedContainer
Here's a very irksome Container
with a given width
, summit
, and color
.
Container ( width: 200 , pinnacle: 200 , color: Colors . scarlet , )
Let's supplant it with AnimatedContainer
and give it a elapsing
:
AnimatedContainer ( width: 200 , tiptop: 200 , color: Colors . red , duration: Duration ( milliseconds: 250 ), )
To animate this, we need to practice a few things:
// one. Convert the parent class to a StatefulWidget class AnimatedContainerPage extends StatefulWidget { @override _AnimatedContainerPageState createState () => _AnimatedContainerPageState (); } grade _AnimatedContainerPageState extends State < AnimatedContainerPage > { // 2. declare the container backdrop equally land variables double _width = 200 ; double _height = 200 ; Color _color = Colors . red ; @override Widget build ( BuildContext context ) { return Scaffold ( torso: Eye ( child: AnimatedContainer ( // 3. laissez passer the land variables equally arguments width: _width , summit: _height , color: _color , duration: Elapsing ( milliseconds: 250 ), ), ), // iv. add a button with a callback floatingActionButton: FloatingActionButton ( child: Icon ( Icons . play_arrow ), onPressed: _update , ), ); } // five. Update the state variables to rebuild the widget void _update () { setState (() { _width = 300 ; _height = 300 ; _color = Colors . green ; }); } }
With the changes above, we tin press the button and the container animates to the new values over the given duration:
Simply if we press the button once more, nothing happens because the state variables are already prepare to the updated values.
To make things more than interesting, we can modify the _update
method to use a random number generator, then that our container animates to a different set of values every time we press the push button.
terminal random = Random (); void _update () { setState (() { _width = random . nextInt ( 300 ). toDouble (); _height = random . nextInt ( 300 ). toDouble (); _color = Colour . fromRGBO ( random . nextInt ( 128 ), random . nextInt ( 128 ), random . nextInt ( 128 ), 1 , ); }); }
Not good enough? Then we can alter the animations curve to modify the rate of change for the animation value:
AnimatedContainer ( width: _width , acme: _height , color: _color , elapsing: Duration ( milliseconds: 250 ), // default curve is Curves.linear curve: Curves . easeInOutCubic , )
This way the animation feels a lot more natural.
Flutter comes with a wide range of curves that you can choose from. And if none of the built-in curves work for yous, you lot can even define your ain
Curve
subclasses.
// a custom sine bend that can be passed to any of the implicitly animated widgets grade SineCurve extends Curve { last double count ; SineCurve ({ this . count = 1 }); @override double transformInternal ( double t ) { return sin ( count * ii * pi * t ) * 0.five + 0.5 ; } }
Alongside AnimatedContainer, Flutter ships with many other implicitly animated widgets such as AnimatedAlign, AnimatedOpacity, AnimatedTheme, and many more than. Here is the total list.
How practise implicitly animated widgets piece of work?
Implicitly animated widgets have 1 or more animatable backdrop that can exist gear up to a target value. When the target value changes, the widget animates the property from the old value to the new value over the given duration.
This makes them easy to use, because all you have to do is to update the target value(s), and the widget volition accept care of the blitheness(s) under the hood.
However, they can simply be used for animations that go forward. If you demand an animation that repeats or goes in reverse, y'all'll demand an explicit animation (see below).
Tweens & TweenAnimationBuilder
Tween stands for in-betwixt, and represents a range with a beginning and an finish:
We tin can use tweens to represent animation values within that range.
And Palpitate gives united states a TweenAnimationBuilder that we tin can use to ascertain our own custom implicit animations.
Use
TweenAnimationBuilder
if you need to create a basic animation, but none of the built-in implicit animations widgets (e.g. AnimatedFoo) does what yous need.
How does this work?
Well, let'southward get back to the boring Container
:
Container ( width: 120 , height: 120 , color: Colors . red )
We tin can wrap this with a TweenAnimationBuilder
and give it a Duration
and a Tween
:
TweenAnimationBuilder < double > ( // 1. add a Duration elapsing: Duration ( milliseconds: 500 ), // two. add together a Tween tween: Tween ( begin: 0.0 , cease: 1.0 ), // three. add a child (optional) kid: Container ( width: 120 , height: 120 , color: Colors . red ), // 4. add together the buiilder builder: ( context , value , kid ) { // 5. apply some transform to the given child return Transform . interpret ( offset: Beginning ( value * 200 - 100 , 0 ), child: child , ); }, )
The
child
argument is optional and can be used for optimization purposes. For more than info, see: Why do TweenAnimationBuilder and AnimatedBuilder have a child statement?
The builder
above gives u.s.a. an animation value inside the range specified by the input Tween
.
In this case, we apply it to interpret the kid widget by value * 200 - 100
on the Ten-axis. This will map animation values between (0, 1)
to an offset between (-100, 100)
.
If nosotros put the lawmaking above inside a new widget form and hot reload, we can see the animation:
At this stage, we tin can extract the Tween'southward finish value to a state variable and use a Slider
to update it:
// 1. utilise a state variable double _value = 0.0 ; // two. pass it to the Tween's terminate value TweenAnimationBuilder < double > ( tween: Tween ( brainstorm: 0.0 , end: _value ), ... ) // 3. Add a slider to update the value Slider . adaptive ( value: _value , onChanged: ( value ) => setState (() => _value = value ), )
This mode, TweenAnimationBuilder
will automatically animate to the new value when we interact with the Slider
:
Other types of Tweens
In the instance above we've used a Tween
of type double
.
But there are several congenital-in Tween subclasses such as ColorTween, SizeTween, and FractionalOffsetTween that yous can utilise to breathing between different colors, sizes, and much more.
If you want, you lot can fifty-fifty ascertain your own
Tween
subclasses. This is useful if you want to breathing betwixt custom objects in your app. Meet the Tween class documentation for more info on this.
AnimationController
If nosotros want to create explicit animations that tin go forwards, in opposite, or even repeat forever, nosotros need an AnimationController
.
Let's meet how to utilize it:
// 1. Define a StatefulWidget subclass grade RotationTransitionPage extends StatefulWidget { const RotationTransitionPage ({ Cardinal ? key }) : super ( key: fundamental ); @override _RotationTransitionPageState createState () => _RotationTransitionPageState (); } class _RotationTransitionPageState extends Country < RotationTransitionPage > // 2. add SingleTickerProviderStateMixin with SingleTickerProviderStateMixin { // three. create the AnimationController belatedly final _animationController = AnimationController ( vsync: this , duration: Duration ( milliseconds: 500 ), ); @override void dispose () { // 4. dispose the AnimationController when no longer needed _animationController . dispose (); super . dispose (); } }
The most interesting line is this:
late terminal _animationController = AnimationController ( vsync: this , duration: Elapsing ( milliseconds: 500 ), );
Past passing
this
to thevsync
argument, we're asking Palpitate to produce a new animation value in sync with the screen refresh charge per unit of our device (ordinarily at 60 frames per second). For more info on this, see: Why Flutter animations demand a vsync/TickerProvider.
If you lot take a lot of widgets with explicit animations, setting up an
AnimationController
every fourth dimension is quite tedious. This article offers two solutions: How to reduce AnimationController average code: Palpitate Hooks vs extending the State class.
AnimatedBuilder
Now that we have our AnimationController
, let's employ it to testify a rotating Container
.
One way to exercise this is to apply an AnimatedBuilder
inside the build()
method:
@override Widget build ( BuildContext context ) { render Scaffold ( body: Middle ( // 1. use an AnimatedBuilder kid: AnimatedBuilder ( // 2. pass our AnimationController equally the animation argument blitheness: _animationController , // 3. pass the child widget that we will animate child: Container ( width: 180 , peak: 180 , color: Colors . red ), // iv. add a builder argument (this volition be called when the animation value changes) architect: ( context , child ) { // v. utilize a Transform widget to utilise a rotation return Transform . rotate ( // 6. the angle is a office of the AnimationController's value angle: 0.5 * pi * _animationController . value , child: child , ); }, ), ), ); }
Still if nosotros run this code, nothing happens. And that's considering we forgot to beginning the animation!
Then we can override the initState()
method and call frontwards()
:
@override void initState () { super . initState (); _animationController . forrard (); }
This will "play" the animation in one case when nosotros load the page (or hot-restart).
If we prefer, we can brand the blitheness repeat forever by calling _animationController.repeat()
instead:
How does AnimatedBuilder work?
Let's revisit this again:
AnimatedBuilder ( // laissez passer our AnimationController as the animation statement animation: _animationController , // pass the child widget that nosotros volition animate child: Container ( width: 180 , height: 180 , color: Colors . red ), // add a builder argument builder: ( context , child ) { // utilise a Transform widget to apply a rotation return Transform . rotate ( // the angle is a function of the AnimationController'due south value bending: 0.five * pi * _animationController . value , child: child , ); }, )
AnimatedBuilder
takes an argument of type Animation<double>
. Since AnimationController
extends Blitheness<double>
, we tin laissez passer it as an argument.
This will crusade the builder
to exist called every time the blitheness value changes. We can employ this to transform the given kid widget, or fifty-fifty return a completely new widget that depends on the blitheness value.
Built-in explicit transition widgets
AnimationController
& AnimationBuilder
are very powerful and you can combine them to create some very custom effects.
But sometimes y'all don't fifty-fifty need an AnimatedBuilder
because Flutter already comes with a ready of born transition widgets that you lot can use.
For example, nosotros can replace all the code above with a RotationTransition
and attain the aforementioned matter with much less attempt:
RotationTransition ( turns: _animationController , kid: Container ( width: 180 , height: 180 , colour: Colors . red ), )
Don't similar rotations? How about a calibration transition?
ScaleTransition ( scale: _animationController , child: Container ( width: 180 , tiptop: 180 , color: Colors . ruddy ), )
With this change nosotros go a scale animation that repeats indefinitely:
AnimationController Listeners
AnimationController
can exercise a lot more than than what we've seen and then far. For instance, we tin can add a condition listener that makes the animation alternate forward and in reverse every time it completes:
@override void initState () { super . initState (); // add a status listener _animationController . addStatusListener (( status ) { if ( status == AnimationStatus . completed ) { _animationController . reverse (); } else if ( condition == AnimationStatus . dismissed ) { _animationController . forward (); } }); // get-go the animation when the widget is first loaded _animationController . frontward (); }
CurvedAnimation
Another cool thing we can do is to employ a Tween
to generate a new Animation
object from the parent AnimationController
. This is oft used to add together a custom animation curve:
tardily final _customAnimation = Tween ( begin: 0.0 , end: 1.0 , ). animate ( CurvedAnimation ( parent: _animationController , curve: Curves . easeInOut , ));
Once nosotros accept this, we tin pass information technology to our transition widget:
ScaleTransition ( // use _customAnimation rather than _animationController every bit an argument scale: _customAnimation , child: Container ( width: 180 , height: 180 , color: Colors . cherry ), )
And by combining the status listener code above with this CurvedAnimation
, we get this:
Implicit vs Explicit animation widgets
Now that we've covered all the basics, we should spot some common patterns in how the Flutter animation APIs are named and used:
Implicitly animated widgets
- they are named
AnimatedFoo
(AnimatedContainer
,AnimatedAlign
etc.) - they take
Duration
andCurve
arguments - they can only breathing forward
- can't find a built-in implicitly animated widget for what you need? Utilize
TweenAnimationBuilder
.
Explicit blitheness widgets
- they are named
FooTransition
(RotationTransition
,ScaleTransition
etc.) - they take an
Animation
argument - they can animate forward, in reverse, or repeat forever
- can't find an explicit animation widget for what you need? Use
AnimatedBuilder
.
What other patterns do these APIs take in common?
- all implicitly blithe widgets extend the same
ImplicitlyAnimatedWidget
parent class. - all explicit transition widgets extend the same
AnimatedWidget
parent grade.
In fact, sometimes information technology's more convenient to define an AnimatedWidget
subclass rather than using AnimatedBuilder
.
For instance, here is how the RotationTransition
widget is implemented within the Flutter SDK:
form RotationTransition extends AnimatedWidget { const RotationTransition ({ Key ? key , required Animation < double > turns , this . alignment = Alignment . center , this . child , }) : super ( key: key , listenable: turns ); // the parent listenable property has type Listenable, // and then we use a getter variable to cast it back to Animation<double> Animation < double > get turns => listenable as Animation < double > ; final Alignment alignment ; final Widget ? child ; // This build method is chosen every time the listenable (blitheness) value changes. // As such, AnimationBuilder is not needed. @override Widget build ( BuildContext context ) { final double turnsValue = turns . value ; final Matrix4 transform = Matrix4 . rotationZ ( turnsValue * math . pi * 2.0 ); return Transform ( transform: transform , alignment: alignment , kid: child , ); } }
In fact, checking the source code for the built-in blitheness widgets is a great mode to learn how to make your own!
sponsor
Lawmaking with Andrea is gratis for anybody. Help me keep it that style by checking out this sponsor:
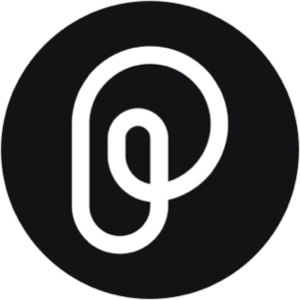
Salvage useful snippets directly from your IDE or browser. Excerpt code from screenshots. Pieces is the fastest style to boost your productivity and stay in period.
Flutter Animations Gallery
There is a lot more to animations than what we take covered. For case:
- how to create staggered animations?
- how to employ a
GestureDetector
to drive the animation of completely custom UI widgets? - how to do blithe theming in Flutter?
To larn more, bank check out my Flutter Animations Gallery on GitHub, which is a showcase all the virtually common animation APIs:
- Palpitate Animations Gallery | GitHub
Official documentation
The Flutter docs have some extensive documentation, codelabs, and tutorials almost the blitheness APIs. Here is the best identify to get started:
- Introduction to animations | Flutter.dev
Palpitate Animations Class
If yous're serious about animations and want to larn how to utilise them in a real-world app, cheque out my consummate Flutter Animations course.
This volition teach you how to build a habit-tracking application with completely custom UI and animations. And it includes 7 hours of in-depth content, full source lawmaking, actress challenges & much more than.
Happy coding!
0 Response to "Animated Penguin Animated Old Fashioned Plane"
Post a Comment